مطالب مرتبط
بخش نظرات این مطلب
تبلیغات
نویسندگان
مطالب تصادفی
- برنامه ای بنویسید که عدد N را خوانده، و مجموع ارقام آن را نشان دهد.
- ذخیره عکس در بانک اطلاعاتی
- تقویم 1393 پیشرفته
- نرم افزار دفتر تلفن هوشمند
- نرم افزار فاکتر گیری از دیتا بیس SQL2008 و c#2010
- پشتیبان گیری و باز یابی بانک اطلاعاتی sql2008در سی شارپ
- تبدیل عدد ریاضی به حروف فارس با سی شارپ 2010
- جدول آنواع داده ها در زبان C
- جدول دسته بندی زبان های برنامه نویسی
- ;کلمات کلیدی در زبان c
- تقدم عملگرها در c
- عملگر های رابطه ای در c
- عملگر های منطقی به ترتیب تقدم در c
- فلوچارت ریشه های یک معادله درجه دوم
- الگوریتم برنامه ای را بنویسید که 10 عدد را گرفته و تعیین کند کدام زوج و کدام فرد است.
- الگوریتم برنامه ای را بنویسید که یک عدد مثبت را خوانده فاکتوریل آن را نمایش دهد.
- الگوریتم برنامه ای را بنویسید که یک عدد را گرفته تعیین کند که آیا کامل است یا خیر؟
- کد تولید رنگ متن تصادفی
- طراحی بنر های تبلیغاتی و لوگو برای سایت و وبلاگ
- اموزش زبان c++ , c
- آموزش زبان c
- آموزش زبان c
ورود کاربران
عضويت سريع
تبادل لینک هوشمند
آخرین نظرات کاربران
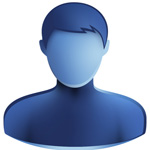
https://www.cialispascherfr24.com/acheter-cialis-pas-che r-belgique/ - 1397/5/25
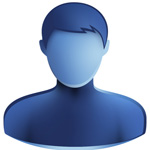
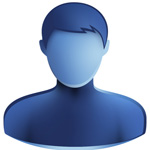
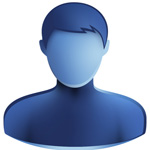
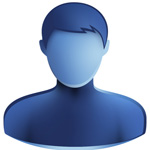
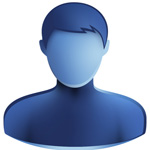
خوبی؟
منو یادته؟
قبلا توی چت لوکس بلاگ صحبت کرده بودیم
چند روزه مشغول جمع آوری بهترین وبلاگهای لوکس بلاگ هستم از تو هم دعوت می کنم با تبادل لینک عضو گروه ما بشی..
مصیب طالبی بهم سر بزن منتظرتم - 1393/10/9
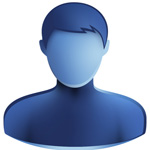
در این روش وبلاگهایی که برای شما نظر میزارن شما برای تشکر به اونا نظر میدین این کار باعث افزایش بازدید وکسب درامد بیشتر میشه چون برای ارسال نظر باید روی وبلاگ شما کلیک کنند و تبلیغات شما نمایش داده میشه وافزایش موجودی .هنگام باز شدن popup اجازه دهید 6 ثانیه باز بمونه تا برای شما ودوستانتون افزایش موجودی داشته باشه .اگر در روز برای 1000 نفر نظر بزارین 1000 بازدید کننده و 6000 تومان درامدخواهید داشت
برای ارسال نظر حتما" لازم نیست یه متن بلند بنویسید هدف کلیک کردن روی وبلاگ دوستتونه یه تشکر خالی کافیه وبا دیدن نظر دوستتون میفهمه شما بودید وبراتون نظر میزاره
من لیست وبلاگهایی که میخوان در این سیستم کار کنن اینجا میزارم برای شروع لینک ارسال نظر پست ثابتتونو برام در قسمت ارسال نظر همین پست بزارین - 1393/10/5
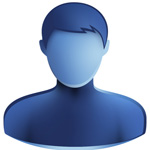
چقدر بگم بیا با من تبادل لینک کن تا بازدید وبلاگ هامون زیاد شه ؟ بیا وبلاگم رو ببین فقط مخصوص افزایش بازدیده . فقط کافیه تبادل لینک کنی. همین الان بیا بهم سر بزن ............. - 1393/10/4
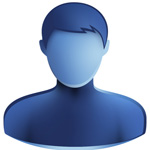
در قسمت موضوعات كليه رشته ها جمع آوري شده است.
فني و مهندسي – علوم انساني – علوم پزشكي - علوم پايه و ....
http://payanname69.lxb.ir
اين هم كد بنر ماست قرار بديد لطفا :
<!-- start logo cod off http://payanname69.loxblog.com --><p align="center"><p align="center"><a href="http://payanname69.loxblog.com" target="_blank"><img border="0" src=" http://8pic.ir/images/nji0kjc0xb78n5qwgh7i.gif" width="468" height="60" alt="دانلود پایان نامه و مقالات اموزشی"></a></p><!--finish logo cod off http://payanname69.loxblog.com -->
- 1393/10/2
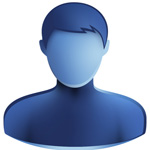
عنوان آگهی شما
توضیحات آگهی در حدود 2 خط. ماهینه فقط 10 هزار تومان
عنوان آگهی شما
توضیحات آگهی در حدود 2 خط. ماهینه فقط 10 هزار تومان
لینک دوستانارسال لینک
- قالب گراف دات آی آر
- بازی خونه
- دانلود کرال
- دانلود فیلم
- سیستم همکاری در فروش
- freebitcoin کسب درآمد با بیت کوین
- زیر چتر خدا
- عشق و علم
- راهنمای گردشگری
- وبلاگ تفریحی و سرگرمی خوشکلا
- خورشید رخا
- هر کی خونده بختش باز شده
- دانلود اهنگ جدید و بسیار زیبای میلاد
- آپلود فایل
- پاندا 2000
- زیاد نخندیا
- حیوانات
- ویروس
- jenifer-lopez
- گزدون
- قلب یخی
- gamer24
- دانلود آهنگ جدید
- مقالات و پروژه های رشته کامپیوتر
- پایه گردان چرخشی خورشیدی
به رایانه چیکاپ امتیاز دهید